import 해주기
import numpy as np
Numpy 배열과 파이썬의 리스트의 성능 차이 비교
import numpy as np
my_arr = np.arange(1000000)
my_list = list(range(1000000))
%time for _ in range(10): my_arr2 = my_arr * 2
%time for _ in range(10): my_list2 = [x * 2 for x in my_list]
CPU times: total: 15.6 ms Wall time: 10.1 ms CPU times: total: 484 ms Wall time: 532 ms |
Numpy를 사용한 코드 순수한 파이썬으로 작성한 코드보다 속도 열배~백배빠르며 메모리 적게 사용
The Numpy ndarray: 다차원배열
import numpy as np
np.random.seed(1)
np.random.randn(2, 3)
array([[ 1.62434536, -0.61175641, -0.52817175], [-1.07296862, 0.86540763, -2.3015387 ]]) |
import numpy as np
np.random.seed(10)
# Generate some random data
data = np.round(np.random.randn(2, 3))*10
data
array([[ 10., 10., -20.], [ -0., 10., -10.]]) |
+ 산술까지 해보면
data*10
array([[ 100., 100., -200.], [ -0., 100., -100.]]) |
data + data
array([[ 20., 20., -40.], [ -0., 20., -20.]]) |
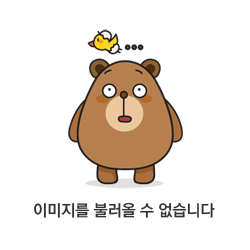
- ndarray는 모두 같은 자료형
- shape: 튜플과 배열에 저장된 각 차원의 크기
- dtype: 튜플과 배열에 저장된 자료형
- ndim: 배열의 차원수
data
data.shape
data.ndim
ndaarays 생성하기
data1 = [6, 8.2, 0, 1]
arr1 = np.array(data1)
arr1
array([6. , 8.2, 0. , 1. ])
data2 = [[1, 2, 3, 4], [5, 6, 7, 8]]
arr2 = np.array(data2)
arr2.ndim
# 2
특수한 배열 만들기
zeros():() 사이즈만큼 0으로 채원진 배열생성
ones():() 사이즈 만큼 1으로 채워진 배열생성
empty(): ()사이즈만큼 빈 배열 생성
np.zeros(10)
# array([0., 0., 0., 0., 0., 0., 0., 0., 0., 0.])
np.zeros((3, 6))
# array([[0., 0., 0., 0., 0., 0.],
# [0., 0., 0., 0., 0., 0.],
# [0., 0., 0., 0., 0., 0.]])
np.empty((2, 3, 2))
"""
array([[[1.49744185e-311, 3.30656698e-315],
[0.00000000e+000, 0.00000000e+000],
[2.22518658e-306, 8.75983079e+164]],
[[7.42862373e-091, 9.76835942e+165],
[4.29228528e-038, 1.09378283e-042],
[3.99910963e+252, 8.34402697e-309]]])
"""
np.arange(15)
#array([ 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14])
ndarrays의 데이터 타입
(1)
arr1 = np.array([1, 2, 3], dtype=np.float64)
print(arr1.dtype)
arr2 = np.array([1, 2, 3], dtype=np.int32)
arr2.dtype
"""
float64
dtype('int32')
"""
(2)
arr = np.array([1, 2, 3, 4, 5])
arr.dtype
"""
dtype('int32')
"""
(3) 정수형을 부동소수점으로 변환
float_arr = arr.astype(np.float64)
float_arr.dtype
"""
dtype('float64')
"""
-> 부동소수정수를 정수형dtype으로 변환하면 소수점 아래자리는 버려짐
arr = np.array([3.7, -1.2, -2.6, 0.5, 12.9, 10.1])
arr
arr.astype(np.int32)
"""
arr = np.array([3.7, -1.2, -2.6, 0.5, 12.9, 10.1])
arr
arr.astype(np.int32)
"""
numeric_strings = np.array(['1.25', '-9.6', '42'], dtype=np.string_)
#numeric_strings.astype(float)
numeric_strings
# array([b'1.25', b'-9.6', b'42'], dtype='|S4')
int_array = np.arange(10) # 0 ~ 9 int64
calibers = np.array([.22, .270, .357, .380, .44, .50], dtype=np.float64) #float64
int_array.astype(calibers.dtype)
"""
array([0., 1., 2., 3., 4., 5., 6., 7., 8., 9.])
"""
empty_uint32 = np.empty(8, dtype='u4')
empty_uint32
"""
array([1, 2, 3, 4, 5, 6, 7, 8], dtype=uint32)
"""
astype을 호출 하면 새로운 dtype과 동일해도 항상 새로운 배열을 생성 (데이터복사)
'Analysis' 카테고리의 다른 글
[데이터마이닝] pandas-taxis 5lab (0) | 2024.12.05 |
---|---|
[데이터마이닝] Scikit-learn Winedata Lab4-sample (0) | 2024.12.05 |
[데이터마이닝] matplotlib - random 3 lap 과제 (0) | 2024.12.05 |
[데이터마이닝] Numpy-학생 SAMPLE upload- Lab2 과제 (1) | 2024.12.05 |
[데이터마이닝] 파이썬 기초(2주차) (0) | 2024.12.05 |